Upload Image From Android Application To server
Uploading image from android application to the server is little bit tough or we can say complex task with comare to the web application because in web application we can directly use the file uploading controls to upload the image from application to the server but in andorid we can upload same as web application but it's not good thing to do that way so we have to convert that image into Base64 string and then upload on the server and server side we have to decode that string into image code using base64 decode
So let's start we have upload image from android to php server
step-1) Create Xml file for desinging
source code
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white"
android:orientation="vertical">
<include layout="@layout/toolbar" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="bottom">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="vertical"
android:id="@+id/linearLayout2">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="horizontal"
android:id="@+id/linearLayout4">
<ImageView
android:id="@+id/img_user"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_margin="5dp"
android:layout_marginLeft="5dp"
android:src="@drawable/unknown_avatar" />
<EditText
android:id="@+id/post_content"
android:layout_width="match_parent"
android:layout_height="200dp"
android:gravity="top|left"
android:padding="10dp"
android:text=""
android:textColorHint="@android:color/darker_gray"
android:background="@android:color/transparent"
android:hint="What's on your mind ?"
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textColor="#424242"
android:textStyle="normal" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="horizontal"
android:id="@+id/linearLayout3">
<ImageView
android:id="@+id/postimages"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:gravity="center_vertical|center_horizontal"
android:layout_alignParentTop="true"
android:layout_below="@+id/linearLayout2"
/>
</LinearLayout>
</LinearLayout>
</RelativeLayout>
<LinearLayout
android:id="@+id/input_bar"
android:layout_width="match_parent"
android:layout_height="90dp"
android:background="@color/grey_bg"
android:orientation="horizontal"
android:padding="5dp">
<android.support.v7.widget.CardView
android:id="@+id/lyt_thread"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginLeft="5dp"
android:layout_weight="1"
android:gravity="center_vertical|center_horizontal"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="5dp"
app:cardElevation="2dp"
app:cardUseCompatPadding="true">
<!-- <EditText
android:id="@+id/text_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white"
android:gravity="top"
android:hint="type message.."
android:padding="10dp" />-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center"
android:gravity="center"
android:orientation="horizontal"
android:weightSum="1">
<ImageView
android:id="@+id/postimage"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center"
android:layout_margin="5dp"
android:layout_weight="0.50"
android:src="@drawable/camera" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
style="@style/FabStyle"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_gravity="center"
android:layout_weight="0.50"
android:clickable="true"
android:src="@drawable/ic_send" />
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
</LinearLayout>
Step:2) Java Source code
Now we where do the code for the selecting image from where we want to uplaod image from gallery or we can caprure image from camera and then set imageinto imageview after converting into the bitmap image so for uploading convert into base64 image string
so create dialog box for selecting which type of image you want to uplaod
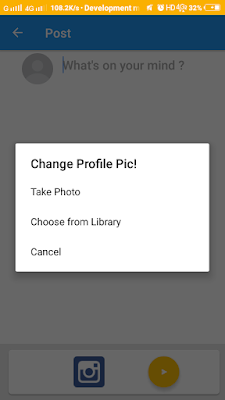
So let's start we have upload image from android to php server
step-1) Create Xml file for desinging
source code
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white"
android:orientation="vertical">
<include layout="@layout/toolbar" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
android:gravity="bottom">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="vertical"
android:id="@+id/linearLayout2">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="horizontal"
android:id="@+id/linearLayout4">
<ImageView
android:id="@+id/img_user"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_margin="5dp"
android:layout_marginLeft="5dp"
android:src="@drawable/unknown_avatar" />
<EditText
android:id="@+id/post_content"
android:layout_width="match_parent"
android:layout_height="200dp"
android:gravity="top|left"
android:padding="10dp"
android:text=""
android:textColorHint="@android:color/darker_gray"
android:background="@android:color/transparent"
android:hint="What's on your mind ?"
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textColor="#424242"
android:textStyle="normal" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="10dp"
android:orientation="horizontal"
android:id="@+id/linearLayout3">
<ImageView
android:id="@+id/postimages"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:gravity="center_vertical|center_horizontal"
android:layout_alignParentTop="true"
android:layout_below="@+id/linearLayout2"
/>
</LinearLayout>
</LinearLayout>
</RelativeLayout>
<LinearLayout
android:id="@+id/input_bar"
android:layout_width="match_parent"
android:layout_height="90dp"
android:background="@color/grey_bg"
android:orientation="horizontal"
android:padding="5dp">
<android.support.v7.widget.CardView
android:id="@+id/lyt_thread"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginLeft="5dp"
android:layout_weight="1"
android:gravity="center_vertical|center_horizontal"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="5dp"
app:cardElevation="2dp"
app:cardUseCompatPadding="true">
<!-- <EditText
android:id="@+id/text_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/white"
android:gravity="top"
android:hint="type message.."
android:padding="10dp" />-->
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center"
android:gravity="center"
android:orientation="horizontal"
android:weightSum="1">
<ImageView
android:id="@+id/postimage"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_gravity="center"
android:layout_margin="5dp"
android:layout_weight="0.50"
android:src="@drawable/camera" />
<android.support.design.widget.FloatingActionButton
android:id="@+id/fab"
style="@style/FabStyle"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_gravity="center"
android:layout_weight="0.50"
android:clickable="true"
android:src="@drawable/ic_send" />
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
</LinearLayout>
Step:2) Java Source code
Now we where do the code for the selecting image from where we want to uplaod image from gallery or we can caprure image from camera and then set imageinto imageview after converting into the bitmap image so for uploading convert into base64 image string
so create dialog box for selecting which type of image you want to uplaod
if (resultCode == RESULT_OK) { if (requestCode == GALLERY_REQUEST) { Uri uri = data.getData(); galleryPhoto.setPhotoUri(uri); String photoPath = galleryPhoto.getPath(); selectedPhoto = photoPath; try { Bitmap bitmap = ImageLoader.init().from(photoPath).getBitmap(); setpostimage.setImageBitmap(bitmap); } catch (FileNotFoundException e) { Toast.makeText(PostGroupData.this, "Something Wrong while choosing photos", Toast.LENGTH_SHORT).show(); } } else if (requestCode == REQUEST_CAMERA) onCaptureImageResult(data);}
private void onCaptureImageResult(Intent data) { Bitmap thumbnail = (Bitmap) data.getExtras().get("data"); ByteArrayOutputStream bytes = new ByteArrayOutputStream(); thumbnail.compress(Bitmap.CompressFormat.JPEG, 90, bytes); File destination = new File(Environment.getExternalStorageDirectory(), System.currentTimeMillis() + ".jpg"); System.out.println("Path...is"+destination); Uri uri = Uri.fromFile(destination); selectedPhoto = uri.getEncodedPath(); System.out.println("Camera Takes snap"+selectedPhoto); FileOutputStream fo; try { destination.createNewFile(); fo = new FileOutputStream(destination); fo.write(bytes.toByteArray()); fo.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } setpostimage.setImageBitmap(thumbnail);}
private void selectImage() { final CharSequence[] items = {"Take Photo","Choose from Library", "Cancel"}; AlertDialog.Builder builder = new AlertDialog.Builder(PostGroupData.this); builder.setTitle("Change Profile Pic!"); builder.setItems(items, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int item) { boolean result = Permission_Proof.checkPermission(PostGroupData.this); if (items[item].equals("Take Photo")) { userChoosenTask = "Take Photo"; if (result) cameraIntent(); } else if (items[item].equals("Choose from Library")) { userChoosenTask = "Choose from Library"; if (result) galleryIntent(); } else if (items[item].equals("Cancel")) { dialog.dismiss(); } } }); builder.show();} private void cameraIntent() { Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(intent, REQUEST_CAMERA);} private void galleryIntent() { Intent i1 = new Intent(Intent.ACTION_PICK, android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI); startActivityForResult(i1, GALLERY_REQUEST); } public String getStringImage(Bitmap bmp){ ByteArrayOutputStream baos = new ByteArrayOutputStream(); bmp.compress(Bitmap.CompressFormat.JPEG, 100, baos); byte[] imageBytes = baos.toByteArray(); String encodedImage = Base64.encodeToString(imageBytes, Base64.DEFAULT); return encodedImage;}
public void postUserData() { progressDialog = ProgressDialog.show(PostGroupData.this, "Please Wait", "Posting Data"); StringRequest stringRequest = new StringRequest(Request.Method.POST, Constraints.BASE_URL, new Response.Listener<String>() { @Override public void onResponse(String response) { System.out.println("Postdata response"+response); progressDialog.dismiss(); try { JSONObject jsonObject = new JSONObject(response); Toast.makeText(getApplicationContext(),"Post Added Successfully",Toast.LENGTH_SHORT).show(); // String strStatus = jsonObject.getString(getString(R.string.key_status)); // String strMessage = jsonObject.getString(getString(R.string.key_message)); // Tools.showSnackbar(mLinearLayoutProfile, strMessage); Intent object = new Intent(PostGroupData.this, GroupDetail.class); startActivity(object); finish(); } catch (JSONException e) { e.printStackTrace(); } } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { progressDialog.dismiss(); } } ) { @Override protected Map<String, String> getParams() throws AuthFailureError { String image = ""; Bitmap bitmap = null; try { bitmap = ImageLoader.init().from(selectedPhoto).getBitmap(); System.out.println("Selected Photo"+bitmap); image = getStringImage(bitmap); } catch (FileNotFoundException e) { e.printStackTrace(); } System.out.println("Image"+image); System.out.println("Image"+image); Map<String,String> jsonParms = new Hashtable<String, String>(); jsonParms.put(getString(R.string.key_mode), "postcontent_grp"); jsonParms.put(getString(R.string.key_userid), UserID); jsonParms.put("postcontent", PostData.getText().toString()); jsonParms.put(getString(R.string.key_image), image); jsonParms.put("grp_id", GroupID); return jsonParms; } }; /*RequestQueue queue = Volley.newRequestQueue(PostDataActivity.this); queue.add(stringRequest);*/ RequestQueue requestQueue = Volley.newRequestQueue(this); requestQueue.add(stringRequest); stringRequest.setRetryPolicy(new DefaultRetryPolicy( 90000, DefaultRetryPolicy.DEFAULT_MAX_RETRIES, DefaultRetryPolicy.DEFAULT_BACKOFF_MULT)); } private String readFileAsBase64String(String path) { try { InputStream is = new FileInputStream(path); ByteArrayOutputStream baos = new ByteArrayOutputStream(); Base64OutputStream b64os = new Base64OutputStream(baos, Base64.DEFAULT); byte[] buffer = new byte[8192]; int bytesRead; try { while ((bytesRead = is.read(buffer)) > -1) { b64os.write(buffer, 0, bytesRead); } return baos.toString(); } catch (IOException e) { Log.e("data", "Cannot read file " + path, e); // Or throw if you prefer return ""; } finally { closeQuietly(is); closeQuietly(b64os); // This also closes baos } } catch (FileNotFoundException e) { Log.e("", "File not found " + path, e); // Or throw if you prefer return ""; } } private static void closeQuietly(Closeable closeable) { try { closeable.close(); } catch (IOException e) { } } /*public String getStringImage(Bitmap bmp){ ByteArrayOutputStream baos = new ByteArrayOutputStream(); bmp.compress(Bitmap.CompressFormat.JPEG, 100, baos); byte[] imageBytes = baos.toByteArray(); String encodedImage = Base64.encodeToString(imageBytes, Base64.DEFAULT); return encodedImage;}*/ private void showFileChooser() { Intent intent = new Intent(); intent.setType("image/*"); intent.setAction(Intent.ACTION_GET_CONTENT); startActivityForResult(Intent.createChooser(intent, "Select Picture"), PICK_IMAGE_REQUEST);}
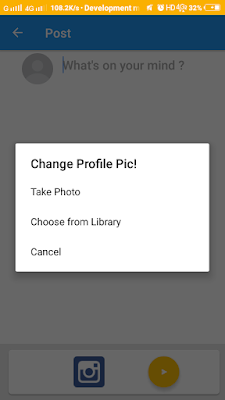
Comments
Post a Comment